Just for clarification, my ents table holds every entity in the game (each one has their own z variable) and is then cycled through to check if the entities have draw functions. If they do, it draws them. The bug seems to be that their drawing function doesn't call properly due to table.sort.
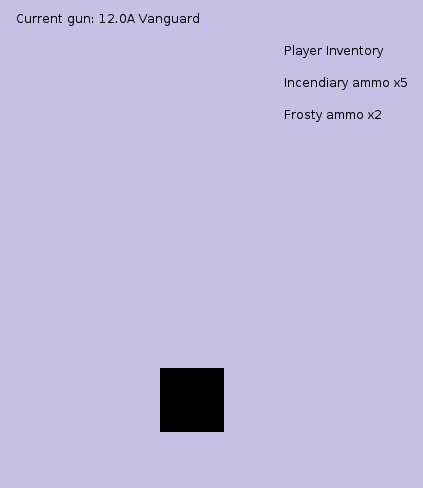
This gif shows my game without any z-ordering. Everything stays stable and never disappears. The bullets have a lifespan, so after a certain amount of frames, they're removed from the ents table, which ceases to draw and update it.
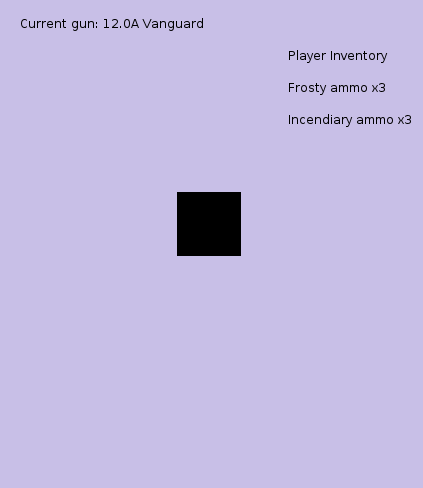
This shows my game with the z-ordering function enabled, sorting the table in a way that makes the entities flash. It seems to do it mostly when the entities delete themselves off-screen.
Here's my draw function, complete with the function I also use for z-ordering.
Code: Select all
draw = function(self, cam)
for k, v in pairs(ents) do
cam:draw(function()
if v.draw ~= nil then
return v:draw()
end
end)
end
if debug.debugMode and debug.debugShow then
love.graphics.setColor(255, 255, 255, 255)
love.graphics.print("FPS: " .. love.timer.getFPS(), 16, 16)
love.graphics.print("Number of entities: " .. entAmt, 16, 32)
return love.graphics.print("Current room: " .. room, 16, 48)
end
end
drawSort = function(a, b)
if a and b then
return a.z > b.z
end
end
The drawSort and draw functions are located in lib\mad\madlib.lua. This is the file that houses the library/engine thing I've been working on for the past few months in my spare time.
Thanks for the help!